Document Finder Tool
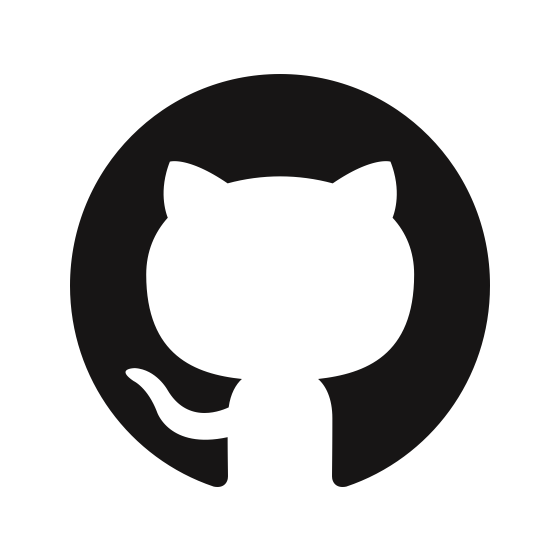
A web application designed to help users discover and access hidden documents, patents, and research papers. The tool uses APIs to tap into various databases ethically.
Tech Stack
- Frontend: HTML, CSS (Bootstrap), JavaScript
- Backend: Python (Flask framework)
- Database: Planned to use AWS services for data storage and management
- Cloud Services: AWS S3 for document storage, AWS Cognito for user authentication and management
Key Features
- User Authentication: User registration, login, and logout using AWS Cognito; Password reset functionality
- Document Management: Upload and search for documents stored in AWS S3; Admin panel for approving or rejecting document upload requests
- Search Functionality: Search tool to find documents based on user input
- Responsive Design: Mobile-friendly design using Bootstrap
Planned Features
- Database Integration: Integration with a database (e.g., AWS RDS) to store metadata and user information
- Advanced Search: Enhanced search capabilities with filters and sorting options
- User Roles: Different user roles (e.g., admin, regular user) with specific permissions
Code Highlights
User Registration Route:
@auth_routes.route('/register', methods=['GET', 'POST'])
def register():
if request.method == 'POST':
email = request.form['email']
password = request.form['password']
name = request.form['name']
try:
response = cognito_client.sign_up(
ClientId=Config.COGNITO_APP_CLIENT_ID,
Username=email,
Password=password,
UserAttributes=[
{'Name': 'email', 'Value': email},
{'Name': 'name', 'Value': name},
{'Name': 'given_name', 'Value': name}
]
)
flash('User registered successfully! Please confirm your email.', 'success')
return redirect('/verify')
except Exception as e:
flash(f"Error during sign-up: {str(e)}", 'danger')
return render_template('register.html')
Admin Control Panel:
<div class="container mt-5">
<h1 class="text-center">Admin Control Panel</h1>
<div class="card mt-4 p-4 shadow-lg">
<h3 class="text-center">Automation Control</h3>
<form method="POST">
<div class="text-center mb-3">
<button type="submit" name="action" value="start_automation" class="btn btn-custom w-100">Start Automation</button>
</div>
<div class="text-center">
<button type="submit" name="action" value="stop_automation" class="btn btn-danger w-100">Stop Automation</button>
</div>
</form>
</div>
</div>
Admin Control Panel
Automation Control
Conclusion
This project demonstrates my ability to work with a full-stack web application, integrating various technologies and cloud services. It showcases your skills in Python, Flask, AWS, and frontend development with HTML, CSS, and JavaScript. Highlighting this project on your portfolio will give potential employers or clients a comprehensive view of your technical capabilities and project management skills.